Node.js 应用程序的自定义检测
默认情况下,APM Insight Node.js 代理会捕获传入的 Web 请求,例如 http、https、http2 和其他类似请求。要获得更多粒度,您可以使用自定义检测 API。这有助于您分析应用程序中的特定事务或代码块。
API 及其功能和语法将在下面介绍。
注意:要在 Node.js 应用程序中使用自定义检测,您必须安装 APM Insight 模块。使用以下命令在您的应用程序中加载 APM Insight 模块:
API 的
监控网页/后台事务:
- 默认情况下,代理会自动收集传入的 Web 请求并显示在 Web 事务选项卡下。但是,代理不监视其他客户端-服务器通信,如套接字连接。可以使用 API 监控此类事务。
- 默认情况下,代理不监视后台事务。您可以通过使用下面的 API 检测后台事务来监控它们。
- 您还可以使用给定的 API 自定义事务的名称或跳过监控的事务。
- 当您检测 Web 或后台事务时,它们必须跟在End transaction API之后。
用于检测 Web 事务的 API
语法
txnName : string value
handler : handler is the function, that will be executed once txn is created
示例
var app = require('express')();
var http = require('http').Server(app);
var io = require('socket.io')(http);
/* this request will be collected automatically*/
app.get('/', function(req, res){
res.sendFile(__dirname + '/index.html');
});
/*need to use custom instrumentation for socket communication*/
io.on('connection', function(socket){
apminsight.startWebTransaction('/message', function(){
doSomething()
........ apminsight.endTransaction();
});
});
http.listen(3000);
输出
用于检测后台事务的 API
语法
txnName : string value
handler : handler is the function, that will be executed once txn is created
示例
function doBackground(){
setInterval( function(){
apminsight.startBackgroundTransaction('routine', function(){
doSomething().........
apminsight.endTransaction();
}
}, 5000);
}
输出
更改/自定义事务名称的 API
语法
cusTxnName : string value
示例
var app = require('express')();
var http = require('http').Server(app);
/* this request will be collected automatically with txn name /admin*/
app.get('/admin', function(req, res){
/* txn name will be changed to /homepage*/
apminsight.setTransactionName('/homepage');
res.sendFile(__dirname + '/index.html');
});
http.listen(3000);
忽略事务的 API
语法
示例
var app = require('express')();
var http = require('http').Server(app);
var io = require('socket.io')(http);
app.get('/logout', function(req, res){
/* this request will be ignored */
apminsight.ignoreCurrentTransaction();
res.sendFile(__dirname + '/index.html');
});
http.listen(3000);
结束事务 API
语法
监控自定义组件
代理捕获默认框架组件、类和方法。但是,只能通过使用以下 API 检测用户定义的类和方法来监视它们。这些详细信息可以在 Traces 选项卡下查看。此外,如果在检测的类或方法中调用任何涉及数据库操作的事务,则这些详细信息将反映在“数据库”选项卡中。
用于跟踪自定义组件的 API
语法
trackerName : string value
componentName : string value
handler : handler is the function, that will be executed
cb : optional param, if it is present then it will be treated as asynchrous tracker
示例 1 - Without cb:
var app = require('express')();
var http = require('http').Server(app);
app.get('/', function(req, res){
apminsight.startTracker('readFile', 'FS', function(){
res.sendFile(__dirname + '/index.html');
});
});
http.listen(3000);
示例 2 - With cb:
var app = require('express')();
var http = require('http').Server(app);
app.get('/', function(req, res){
apminsight.startTracker('readFile', 'FS', function(cb){
doSomething()......
cb();
}, function(){
// send response
});
});
http.listen(3000);
输出

跟踪处理的错误
通常,代理会捕获所有异步 i/o 错误和未处理的错误。此 API 可用于处理错误。例如,如果您使用 try-catch 方法处理了错误,则可以通过此 API 通知此类错误,并且通知的错误与其对应的事务相关联。可以在错误和跟踪选项卡下查看捕获的错误。
用于跟踪已处理错误的 API
语法
err : Error object
示例
var app = require('express')();
var http = require('http').Server(app);
/* this request will be collected automatically*/
app.get('/', function(req, res){
try{
fetchAndSendResponse();
}catch(err){
apminsight.trackError(err)
sendErrorResponse();
}
});
http.listen(3000);
输出
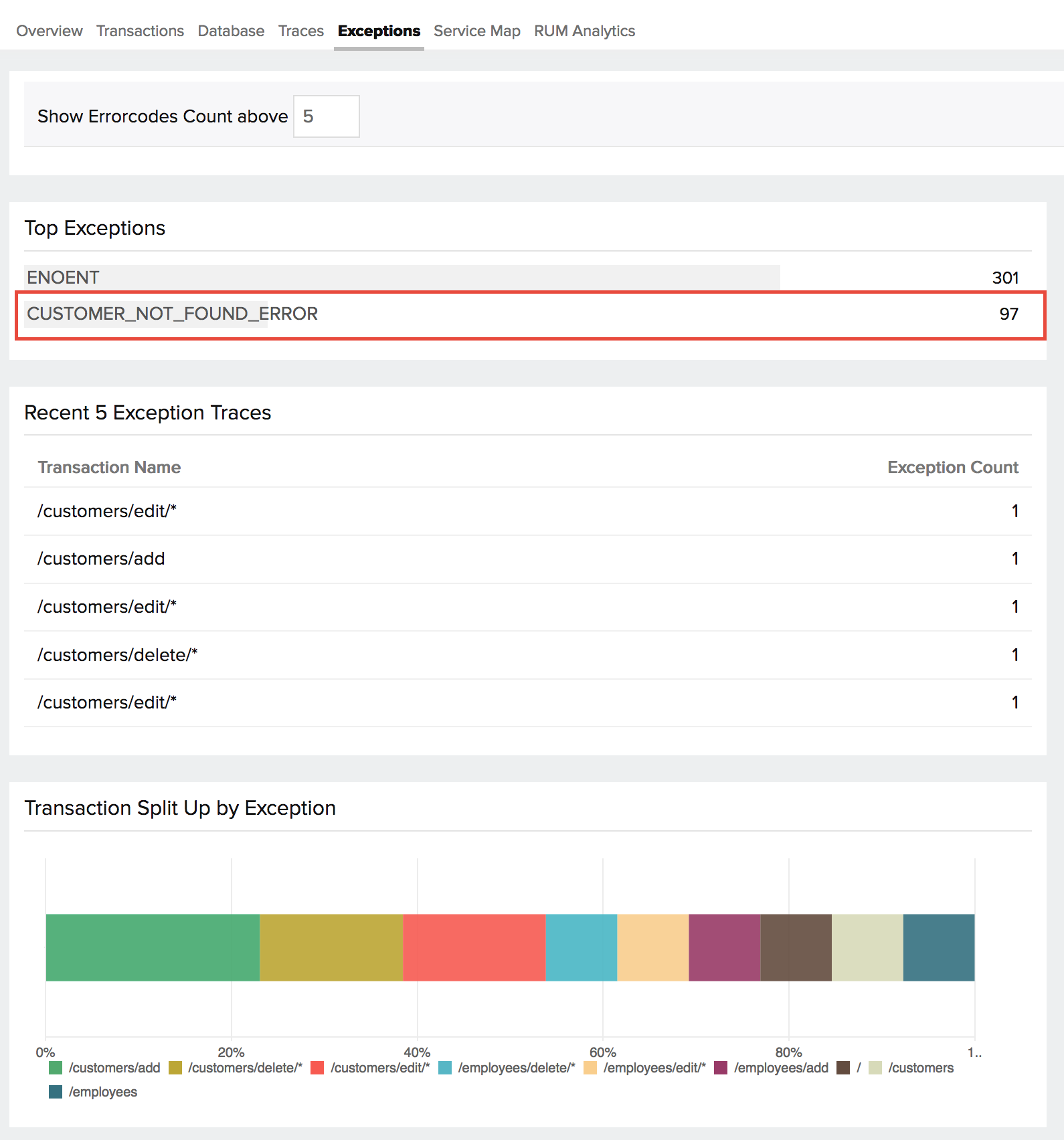
自定义应用参数
使用 App 参数,您可以监控重要参数,例如变量的大小和频率或应用程序中的操作。要了解 App 参数的工作原理,请参阅此处。
用于检测应用程序参数的 API
incrementCustomMetric: incrementCustomMetric: 此 API 收集自定义指标的总和。
语法
metricName : string value
metricValue : optional, if it is not present then metric will incremented by 1
示例
var app = require('express')();
var http = require('http').Server(app);
app.get('/buy', function(req, res){
apminsight.incrementCustomMetric('products', req.product.count);
res.sendFile(__dirname + '/index.html');
});
http.listen(3000);
averageCustomMetric: 此 API 收集自定义指标的平均值。
语法
metricName : string value
metricValue : number
示例
var app = require('express')();
var http = require('http').Server(app);
app.get('/pay', function(req, res){
apminsight.averageCustomMetric('amount', req.amount);
res.sendFile(__dirname + '/index.html');
});
http.listen(3000);
输出
跟踪自定义参数
要为跟踪赋予上下文意义,您可以添加其他参数来帮助您识别事务跟踪的上下文。 上下文度量可以是任何东西,会话 ID、用户 ID 或某些方法参数,它们可以帮助您识别有关事务跟踪的特定信息。 一个事务跟踪最多可以添加 10 个参数,这些参数可以在跟踪汇总下查看
跟踪自定义参数的语法
apminsight.addParameter("key", value);
Key - 参数名称
Value - 值的参数。它可以是任何类型,内部代理将它们转换为字符串。
示例
var apminsight = require('apminsight');
var app = require('express')();
var http = require('http').Server(app);
app.get('/', function(req, res){
apminsight.addParameter("User Detail", "APM User");
apminsight.addParameter("User ID", 408);
res.sendFile(__dirname + '/index.html');
});
http.listen(3000);
输出:
添加的参数值将显示在跟踪详情选项卡的自定义参数部分下,如下所示: